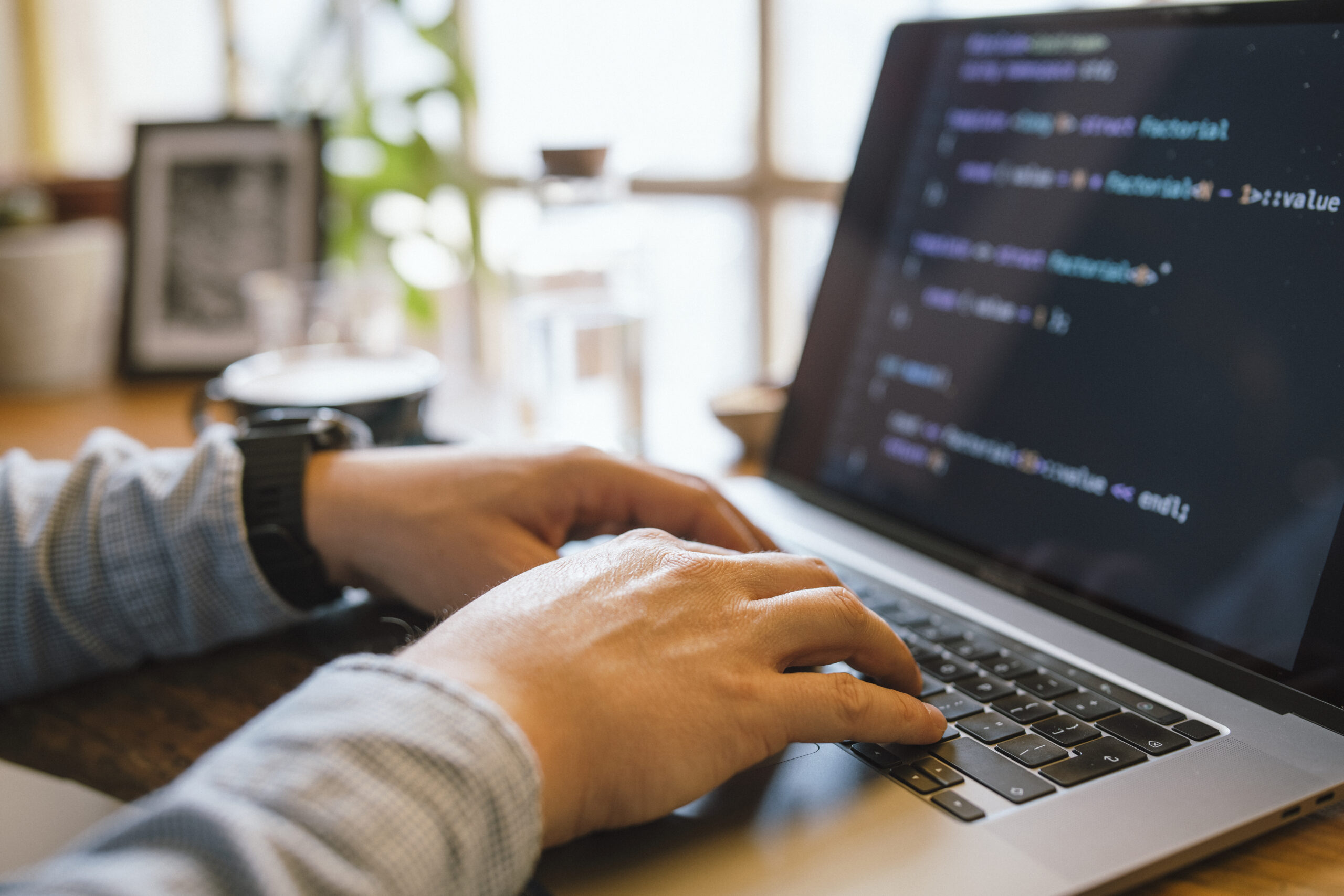
Debugging is One of the more crucial — nonetheless often ignored — capabilities in a very developer’s toolkit. It isn't really pretty much correcting damaged code; it’s about understanding how and why issues go Improper, and Finding out to Consider methodically to resolve troubles successfully. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of stress and substantially increase your productiveness. Listed below are numerous methods to assist builders amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
One of many quickest means builders can elevate their debugging capabilities is by mastering the equipment they use each day. While crafting code is just one Section of improvement, knowing ways to communicate with it efficiently throughout execution is Similarly critical. Contemporary enhancement environments appear equipped with impressive debugging capabilities — but many builders only scratch the surface of what these resources can perform.
Just take, for instance, an Built-in Advancement Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources assist you to established breakpoints, inspect the value of variables at runtime, action via code line by line, and perhaps modify code within the fly. When used accurately, they let you notice precisely how your code behaves all through execution, which can be a must have for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-end developers. They allow you to inspect the DOM, keep an eye on community requests, check out serious-time efficiency metrics, and debug JavaScript during the browser. Mastering the console, sources, and network tabs can convert irritating UI troubles into workable tasks.
For backend or program-stage builders, resources like GDB (GNU Debugger), Valgrind, or LLDB offer you deep control above jogging procedures and memory management. Understanding these instruments can have a steeper learning curve but pays off when debugging functionality difficulties, memory leaks, or segmentation faults.
Beyond your IDE or debugger, turn out to be cozy with Model Regulate systems like Git to know code historical past, uncover the precise instant bugs were being introduced, and isolate problematic modifications.
In the long run, mastering your applications means going beyond default settings and shortcuts — it’s about creating an intimate understanding of your advancement surroundings to ensure when troubles occur, you’re not missing in the dead of night. The higher you already know your instruments, the greater time it is possible to commit fixing the actual issue rather than fumbling through the procedure.
Reproduce the condition
One of the more significant — and sometimes neglected — measures in successful debugging is reproducing the issue. Prior to leaping in the code or generating guesses, developers need to produce a regular setting or scenario where the bug reliably seems. With no reproducibility, fixing a bug results in being a video game of possibility, frequently bringing about wasted time and fragile code modifications.
The initial step in reproducing a difficulty is gathering just as much context as you possibly can. Ask issues like: What actions triggered The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or mistake messages? The more element you might have, the simpler it results in being to isolate the exact disorders beneath which the bug occurs.
As you’ve collected more than enough data, try to recreate the situation in your local setting. This could indicate inputting the same knowledge, simulating similar consumer interactions, or mimicking procedure states. If the issue seems intermittently, consider composing automatic tests that replicate the edge scenarios or state transitions concerned. These assessments not only aid expose the condition but additionally protect against regressions Down the road.
At times, The problem may very well be atmosphere-distinct — it'd happen only on specific running units, browsers, or under certain configurations. Employing applications like virtual machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the condition isn’t just a stage — it’s a attitude. It calls for endurance, observation, in addition to a methodical approach. But when you can constantly recreate the bug, you are previously midway to repairing it. By using a reproducible circumstance, You may use your debugging applications more effectively, test possible fixes safely, and communicate more clearly with your team or users. It turns an summary criticism right into a concrete problem — and that’s the place developers thrive.
Study and Realize the Error Messages
Mistake messages will often be the most beneficial clues a developer has when a thing goes Erroneous. As an alternative to viewing them as irritating interruptions, developers should really study to deal with error messages as immediate communications with the technique. They usually tell you what precisely took place, in which it happened, and in some cases even why it took place — if you understand how to interpret them.
Start by examining the concept cautiously As well as in entire. Numerous builders, particularly when under time tension, glance at the first line and promptly commence making assumptions. But further within the mistake stack or logs might lie the legitimate root lead to. Don’t just copy and paste error messages into search engines like yahoo — browse and recognize them first.
Split the mistake down into areas. Can it be a syntax error, a runtime exception, or simply a logic error? Will it stage to a certain file and line quantity? What module or purpose triggered it? These inquiries can guide your investigation and position you towards the accountable code.
It’s also handy to know the terminology with the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java normally adhere to predictable designs, and Discovering to recognize these can considerably increase your debugging method.
Some glitches are obscure or generic, As well as in Those people instances, it’s critical to look at the context in which the error transpired. Test related log entries, input values, and recent alterations during the codebase.
Don’t overlook compiler or linter warnings either. These typically precede larger sized issues and provide hints about prospective bugs.
Eventually, mistake messages are usually not your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, aiding you pinpoint troubles speedier, cut down debugging time, and turn into a more efficient and assured developer.
Use Logging Properly
Logging is Just about the most strong instruments in a very developer’s debugging toolkit. When made use of correctly, it offers serious-time insights into how an software behaves, serving to you have an understanding of what’s going on underneath the hood while not having to pause execution or action from the code line by line.
A fantastic logging tactic commences with being aware of what to log and at what degree. Frequent logging amounts contain DEBUG, Information, WARN, Mistake, and Deadly. Use DEBUG for thorough diagnostic details in the course of improvement, INFO for typical situations (like prosperous start off-ups), WARN for potential challenges that don’t split the appliance, ERROR for precise troubles, and FATAL when the process can’t keep on.
Stay away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure crucial messages and decelerate your process. Give attention to key situations, condition changes, enter/output values, and significant selection details with your code.
Format your log messages Plainly and constantly. Include context, for instance timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re In particular beneficial in generation environments exactly where stepping by code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about balance and clarity. Using a perfectly-believed-out logging technique, you can reduce the time it will require to identify problems, achieve further visibility into your purposes, and improve the Total maintainability and trustworthiness of your code.
Believe Just like a Detective
Debugging is not simply a technological job—it's a kind of investigation. To proficiently identify and repair bugs, developers have to tactic the procedure like a detective solving a mystery. This attitude will help stop working elaborate issues into manageable components and stick to clues logically to uncover the basis lead to.
Start off by accumulating proof. Think about the signs or symptoms of the condition: mistake messages, incorrect output, or performance issues. Just like a detective surveys a crime scene, collect as much relevant information as you are able to with out jumping to conclusions. Use logs, test cases, and person experiences to piece alongside one another a transparent photo of what’s taking place.
Up coming, type hypotheses. Question by yourself: What may be leading to this conduct? Have any modifications recently been made into the codebase? Has this challenge transpired just before below similar instances? The target is usually to narrow down possibilities and detect likely culprits.
Then, check your theories systematically. Attempt to recreate the condition in a very controlled environment. When you suspect a particular function or part, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, check with your code inquiries and let the effects direct you closer to the reality.
Spend shut consideration to little facts. Bugs usually hide while in the least predicted locations—similar to a missing semicolon, an off-by-1 mistake, or even a race ailment. Be extensive and patient, resisting the urge to patch The problem without thoroughly comprehending it. Non permanent fixes could disguise the real challenge, only for it to resurface later on.
Last of all, preserve notes on Anything you attempted and figured out. Just as detectives log their investigations, documenting your debugging method can help you save time for potential challenges and assist Many others comprehend your reasoning.
By wondering like a detective, developers can sharpen their analytical techniques, approach difficulties methodically, and develop into more effective at uncovering hidden concerns in advanced systems.
Create Exams
Producing checks is one of the most effective approaches to transform your debugging competencies and overall advancement effectiveness. Assessments not simply assistance capture bugs early but also serve as a safety net that gives you self-assurance when generating improvements in your codebase. A properly-examined software is simpler to debug as it means that you can pinpoint particularly where by and when a dilemma takes place.
Get started with device assessments, which center on particular person capabilities or modules. These smaller, isolated assessments can speedily expose no matter whether a certain piece of logic is Operating as expected. When a test fails, you immediately know where by to glimpse, noticeably cutting down enough time put in debugging. Unit tests are Primarily handy for catching regression bugs—difficulties that reappear soon after Formerly being preset.
Upcoming, integrate integration tests and close-to-conclusion assessments into your workflow. These assist ensure that many portions of your application work jointly easily. They’re particularly practical for catching bugs that arise in complicated units with a number of elements or products and services interacting. If anything breaks, your tests can show you which Portion of the pipeline unsuccessful and beneath what circumstances.
Producing exams also forces you to definitely Feel critically regarding your code. To test a element effectively, you need to website be aware of its inputs, expected outputs, and edge scenarios. This degree of knowledge Normally potential customers to better code framework and much less bugs.
When debugging a problem, crafting a failing check that reproduces the bug is often a powerful initial step. When the test fails persistently, you can give attention to correcting the bug and watch your examination go when the issue is settled. This technique makes certain that exactly the same bug doesn’t return Sooner or later.
To put it briefly, writing exams turns debugging from the disheartening guessing sport into a structured and predictable course of action—encouraging you catch a lot more bugs, speedier plus more reliably.
Consider Breaks
When debugging a difficult situation, it’s uncomplicated to be immersed in the problem—looking at your display for hrs, striving Option just after solution. But Just about the most underrated debugging applications is solely stepping absent. Having breaks helps you reset your mind, decrease aggravation, and often see the issue from a new perspective.
When you're too close to the code for too lengthy, cognitive fatigue sets in. You may begin overlooking obvious errors or misreading code that you simply wrote just hours before. During this point out, your Mind gets considerably less productive at difficulty-solving. A short wander, a espresso split, or perhaps switching to a different task for ten–quarter-hour can refresh your emphasis. Several developers report finding the foundation of a challenge once they've taken time to disconnect, permitting their subconscious get the job done while in the track record.
Breaks also help reduce burnout, In particular for the duration of for a longer time debugging sessions. Sitting down in front of a monitor, mentally caught, is not just unproductive but will also draining. Stepping absent permits you to return with renewed energy and also a clearer attitude. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
In the event you’re trapped, an excellent general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–10 moment crack. Use that time to maneuver close to, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, especially beneath limited deadlines, nonetheless it actually contributes to faster and simpler debugging Ultimately.
In a nutshell, having breaks isn't an indication of weak spot—it’s a smart approach. It gives your brain Place to breathe, increases your viewpoint, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Each bug you come across is a lot more than simply a temporary setback—It really is a chance to mature as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or simply a deep architectural problem, each can train you a thing valuable in the event you make time to replicate and review what went wrong.
Begin by asking oneself a number of critical thoughts once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with far better procedures like unit screening, code testimonials, or logging? The solutions typically expose blind spots within your workflow or being familiar with and assist you Establish much better coding patterns going ahead.
Documenting bugs can even be an outstanding practice. Retain a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and what you learned. Over time, you’ll begin to see designs—recurring troubles or frequent errors—that you could proactively avoid.
In workforce environments, sharing Anything you've figured out from a bug together with your friends is often Specially effective. Whether or not it’s via a Slack concept, a short generate-up, or A fast understanding-sharing session, helping Some others stay away from the exact same difficulty boosts staff efficiency and cultivates a much better Finding out culture.
Additional importantly, viewing bugs as lessons shifts your mindset from annoyance to curiosity. As opposed to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. All things considered, some of the finest developers are certainly not the ones who produce ideal code, but people that constantly study from their errors.
In the long run, Every bug you deal with adds a whole new layer to your ability established. So next time you squash a bug, take a instant to reflect—you’ll arrive absent a smarter, more capable developer thanks to it.
Conclusion
Strengthening your debugging competencies will take time, exercise, and patience — nevertheless the payoff is big. It will make you a more productive, self-assured, and able developer. The next time you are knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become superior at Anything you do.